My Journey Of Building Graphics Rendering Engine Using Vulkan SDK | Week 9
Just one more week before final presentation. This week, I worked solely on Shadow Mapping & proxy model pipeline as I dived into it and still not able to get desired result.
Howdy Everyone! If you’re regular visitor and here to see my progress on this project, I am glad to see you here. If you’re new visitor, You might like to start from my first blogpost when I started working on this project from scratch. Thanks for visiting.
So Lets move on to Shadow Mapping. Shadow mapping follows the same principle of Projective Texture mapping with Occlusion testing.
- First we render object from the perspective of light to generate depth / shadow map.
- Then we render the scene from the perspective of camera and use your generated depth map to determine if an object is in Shadow or not.
One of the important thing to note that is for shadow map, we are rendering scene from the perspective of light to the offscreen buffer. So it’s gonna require different pipeline than what we already have.
We are using simplest vertex shader as a shader input to our offscreen pipeline. It has one uniform buffer where we are giving WorldLightProjectionMatrix as an input. We don’t need fragment shader for this pipeline so we have excluded it. You can observe that vertex shader depthMap.vert in Shaders directory.
As we are passing one uniform buffer in our offscreen pipeline, we have created new uniform buffer called OffscreenUniformBufferObjectVS which is associated with its own staging buffer and memory. To create new pipeline, we are reusing most of the model pipeline declarations but we have few additional changes like have enabled depth bias, attached our depthMap vertex shader, new render pass, new descriptor set layout and no color blending attachments.
Lets look into render pass. If you can recall my post about Imgui integration, you are aware that we used same render pass for rendering ImGui but here, we are gonna use different render pass to render scene from view of light. One important thing to note is, this new renderpass doesn’t have any color attachments. We only have depth attachment along with depth reference.
Once we create shadow map, we need to pass it to our main pipeline fragment shader for depth comparison. for that, we need to create new Image sampler along with Image view and its associated buffer and memory. Creating sampler is easy like the same way we created Texture sampler for model or Projected Texture Sampler. We are using CLAMP_TO_EDGE address mode along with Linear Filter & Opaque white border.
In our depth attachment’s description, for store operation (storeOp) we are storing depth content at the end of subpass where its last used. We are using 2 subpass dependencies to handle layout transitions. Next, we have created offscreen frame buffer for ShadowMap. where we have only one attachment which is shadowMapImageView.
Next thing is we need to create Descriptor set layout binding for our shadow map image sampler. We are using the same model pipeline layout information for creating descriptor set layout. We have also added additional entries in our Descriptor Pool for Combined Image Sampler and Uniform Buffer. Now we have set up descriptor Pool , we can set up Descriptor Sets . We only need one descriptor set mainly for our Uniform buffer which we are passing in our offscreen pipeline vertex shader.
btw, Feel free to visit my GitHub repository to checkout my project and download code files.
Link to This Project’s GitHub Repository
You can go to “releases” section on my GitHub repo and download zip file under tag “Week_IX_Progress“. So You can look into the code which I’m referencing here.
We have created graphics pipeline to render scene from light’s point of view into offscreen buffer. but we still haven’t added any model which we are going to use to cast shadow on our home. Currently we have only chalet model in our scene which isn’t sufficient for shadow mapping. We also need to add proxy models for better visualization. For this, we need to create another pipeline which we will call as proxyModelPipeline.
As we are creating new pipeline, it comes with whole set of definitions like what we have seen before. It includes Pipeline Layout, Descriptor Set Layout, Descriptor sets, Uniform buffers with mapped memories.
In new pipeline we are going to attach 2 new shaders called as ProxyModelVert & proxyModelFrag . Both are simple shaders. Vertex shader takes ModelViewProjection as an input and passes color to fragment shader which simply outputs color. This pipeline structure is very identical to our model pipeline so we are re-using model pipeline structure.
As usual, we have added new uniform buffer entry in descriptor pool and created descriptor set layout and sets. We only need one uniform buffer for passing ModelViewProjection information.
In this pipeline, we are using the same render pass as our model pipeline as both pipelines are identical. For updating offscreen uniform buffer, I’ve added new method called updateUniforBufferOffscreen() which calculate WorldLightViewProjection matrix using light’s pov. One important thing to note is, light behaves the same way like camera but its offscreen so I’ve instantiated another camera called mShadowLight which I’ve translated and rotated to desired location and we are using it’s Projection matrix, position & up vector calculate ViewProjection matrix which is multiplied with model matrix to produce WorldLightViewProjection matrix.
If you did everything correctly, you should get following output for shadow mapping.
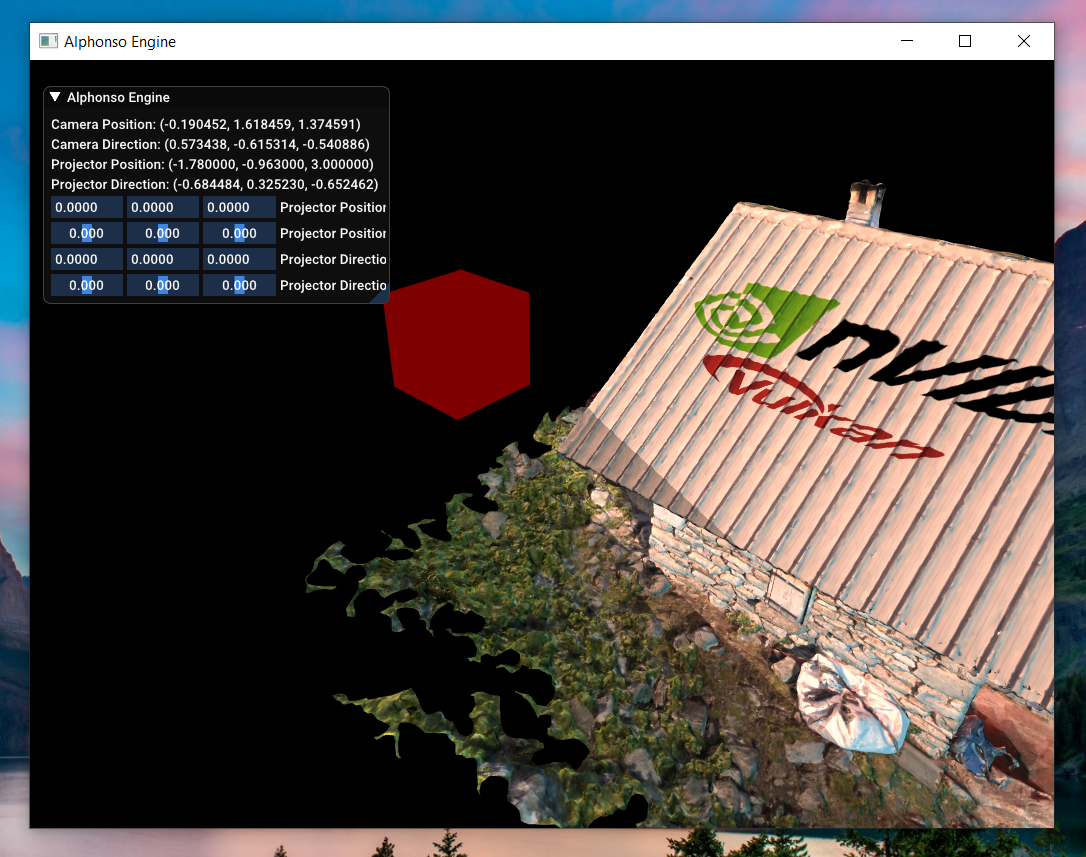
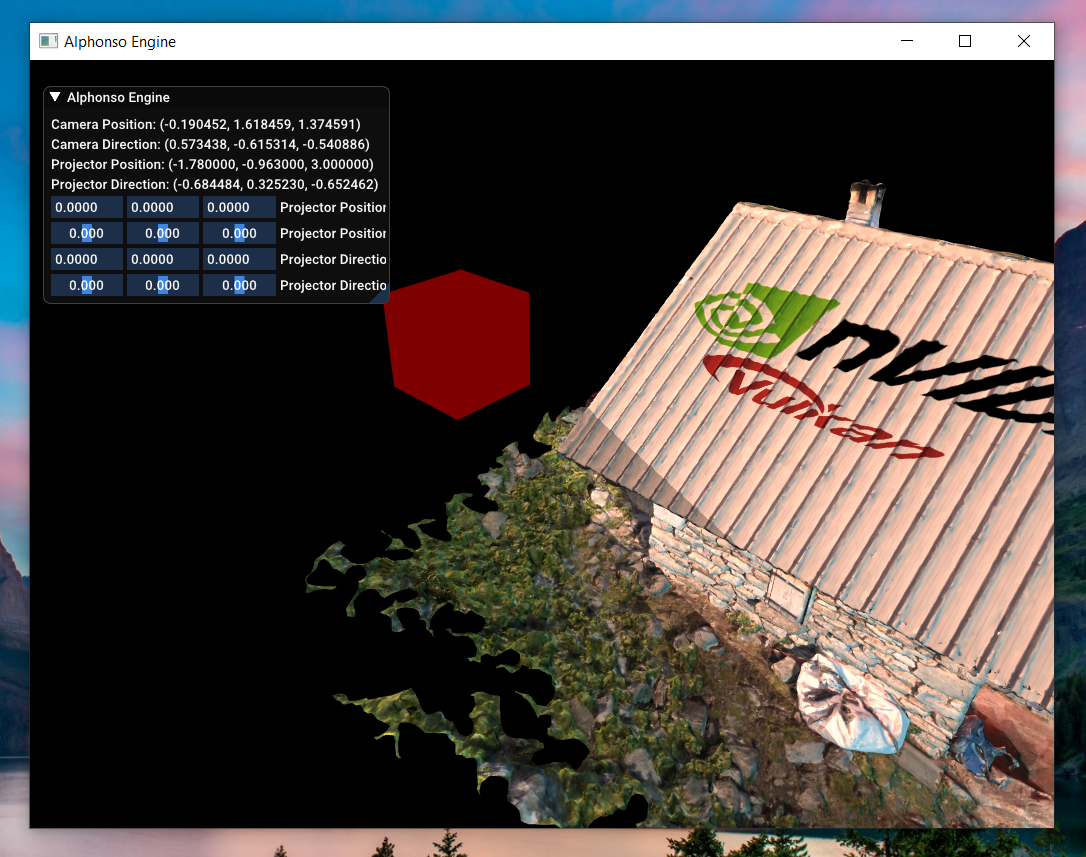
But this shadow doesn’t look right as its incorrect. So I did run my program through RenderDoc as well as Nvidia Nsight to check debug output, Here’s my output through Nvidia Nsight.
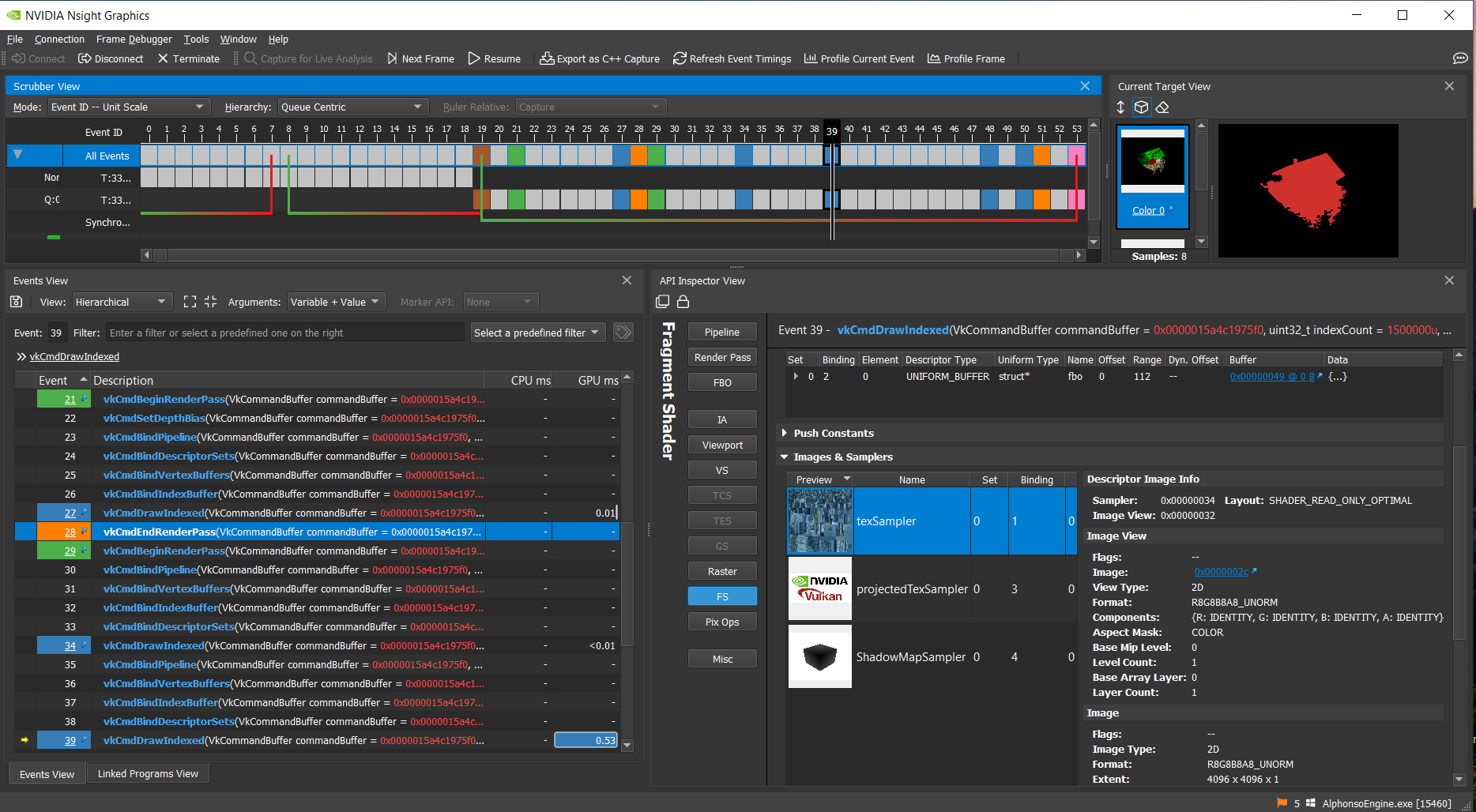
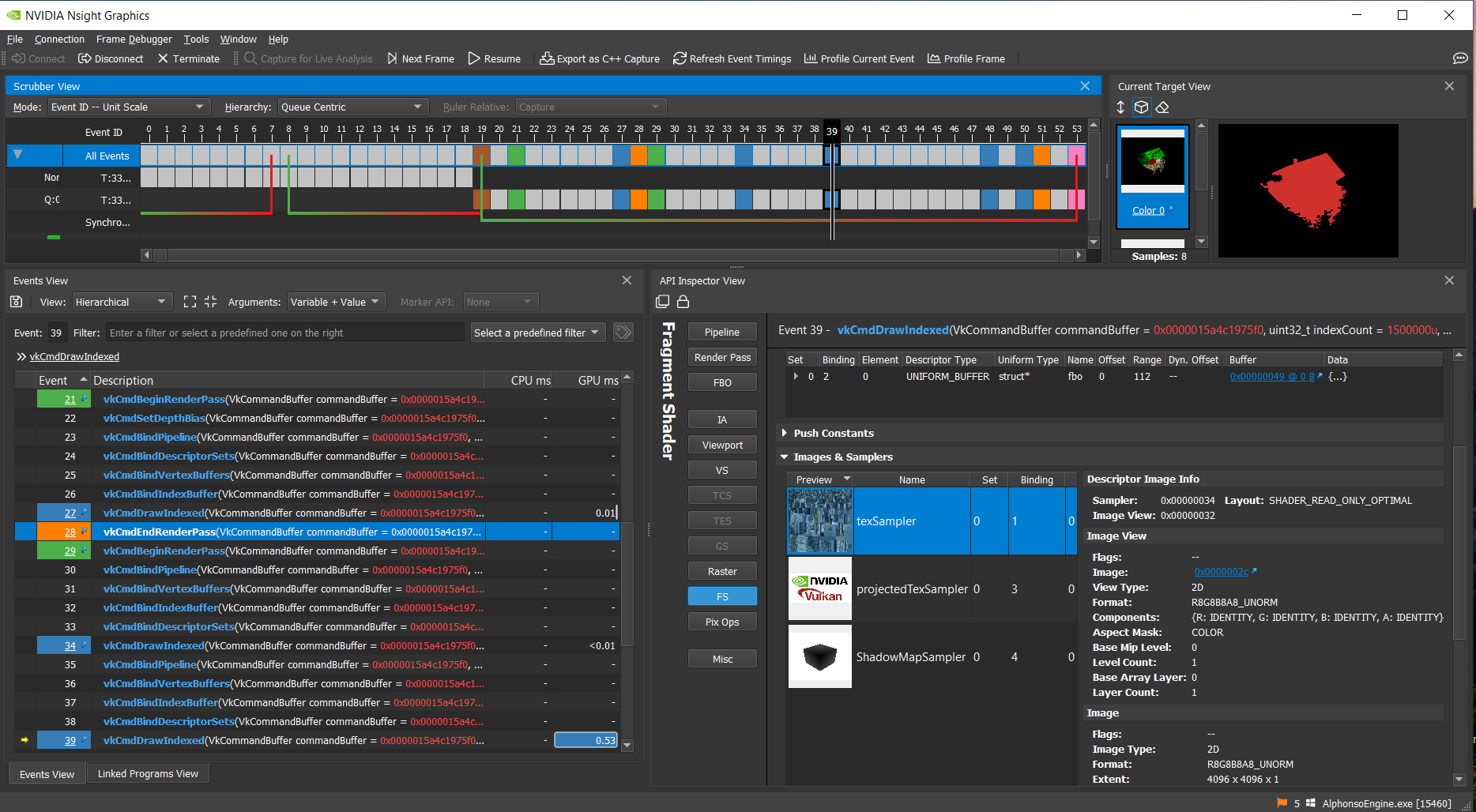
So if you look closely, you can see that Nsight shows my shadow map sampler getting passed correctly to fragment shader. so it means there is problem in fragment shader when we create shadow texture coordinates. I tried debugging it but haven’t find any solution yet. Also both RenderDoc and Nsight doesn’t support shader debugging for Vulkan so its bit difficult to debug at this moment. This is what have for this week.
Next Week Task:-
Color filtering is the pending task after shadow mapping. If I could able to finish shadow mapping issues, I will try to move onto Color filtering.
Thanks for visiting. Have a great day ahead.. 🙂